Choosing the right way to get data from MongoDB can make or break your Node.js application. If you’re using Mongoose, you’ve got two main options: regular queries (like find()
) or the aggregation pipeline. But Mongoose Query Syntax vs Aggregation Pipeline which one should you pick for your project?
This guide breaks down both approaches in simple terms, showing you real examples that you can actually use in your code today. We’ll look at what makes each option good (or not so good) for different situations, and help you make smart choices for your app.
What Are Mongoose Queries and Aggregation Pipelines?
Before we dive deep, let’s get clear on what we’re talking about:
Mongoose queries are the straightforward way to get data. Think of them like asking simple questions: “Find me all users named John” or “Get me the newest blog posts.” You use methods like find()
, findOne()
, and findById()
.
Aggregation pipelines are more like assembly lines for your data. The database processes your information through different stages, transforming it as it goes. You might filter, group, calculate totals, or reshape your data – all in a single request to MongoDB.
Let’s look at what makes each one tick.
Mongoose Query Syntax: The Familiar Approach
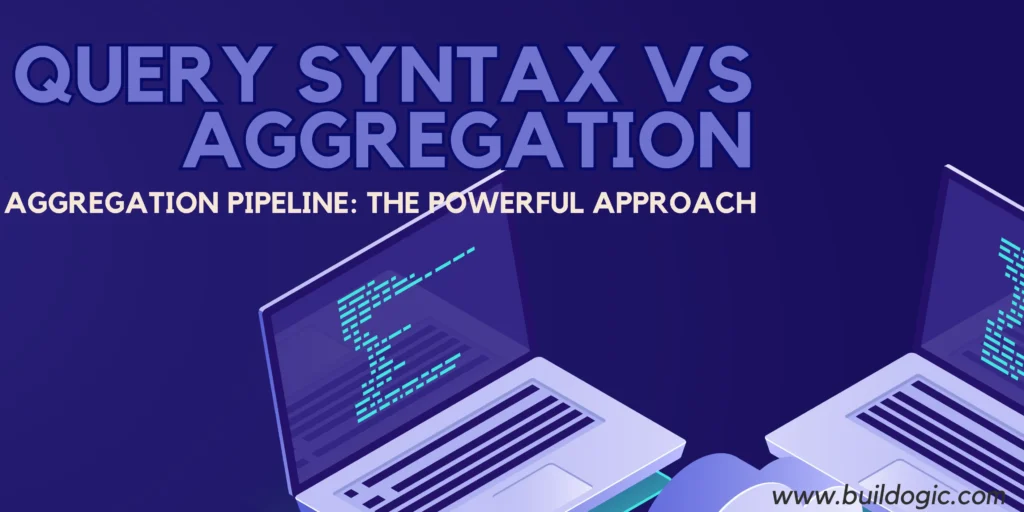
How Regular Queries Work
If you’ve used Mongoose before, you’re probably familiar with this pattern:
// Finding active users, sorted by name
User.find({ status: 'active' })
.sort('name')
.limit(10)
.exec()
.then(users => {
// Do something with the users
});
This is easy to understand – you’re getting users whose status is “active”, sorting them by name, and limiting the results to 10 users.
You can chain methods to build up more complex queries:
// Find users over 21 years old, sort by age, and select only name and email
User.find()
.where('age').gt(21)
.sort('-age')
.select('name email')
.exec()
When to Use Regular Queries
Regular queries work great when:
- You need simple CRUD operations (Create, Read, Update, Delete)
- You’re working with a single collection
- Your data filtering needs are straightforward
- You want to retrieve complete documents
- You need to use Mongoose’s document methods and validation
For example, if you’re building a blog and need to get all posts by a certain author, sorted by date, a regular query is perfect:
Post.find({ author: authorId })
.sort('-createdAt')
.populate('author')
.exec()
Pros of Regular Queries
- Easy to read and write – The syntax feels natural and flows well
- Works with Mongoose models – Returns full Mongoose documents with all their methods
- Good for simple operations – Handles basic data retrieval without fuss
- Leverages indexes well – MongoDB optimizes these queries nicely
Cons of Regular Queries
- Limited data processing – Can’t do calculations or transformations on the database side
- May require multiple queries – Complex operations might need several round-trips to the database
- No built-in grouping – Can’t easily count, sum, or average data without extra code
Aggregation Pipeline: The Powerful Approach
How the Pipeline Works
The aggregation pipeline is like a factory line for your data. Each stage transforms the data before passing it to the next:
Order.aggregate([
{ $match: { status: 'completed' } },
{ $group: {
_id: '$productId',
totalSold: { $sum: '$quantity' }
}},
{ $sort: { totalSold: -1 } }
])
This pipeline:
- Finds all completed orders
- Groups them by product ID
- Adds up the quantities for each product
- Sorts products by total sales (highest first)
The result is a list of products with their total sales – all in one database call.
When to Mongoose Query Syntax vs Aggregation Pipeline
Aggregation shines when:
- You need to transform or calculate data
- You want to join information from multiple collections
- You need to group data and run calculations (sums, averages, etc.)
- You want to minimize the amount of data sent to your application
- You’re building reports or analytics
For example, if you’re creating a dashboard that shows sales by product category, aggregation is your best bet:
Order.aggregate([
{ $match: { orderDate: { $gte: new Date('2023-01-01') } } },
{ $lookup: {
from: 'products',
localField: 'productId',
foreignField: '_id',
as: 'product'
}},
{ $unwind: '$product' },
{ $group: {
_id: '$product.category',
totalSales: { $sum: '$totalAmount' }
}},
{ $sort: { totalSales: -1 } }
])
Pros of Aggregation
- Powerful data processing – Can transform, calculate, and reshape data
- Single database call – Combines multiple steps into one request
- Reduced network traffic – Only returns the data you actually need
- Database-side processing – Leverages MongoDB’s processing power
Cons of Aggregation
- More complex syntax – Takes longer to learn and can be harder to read
- Returns plain objects – Results aren’t Mongoose models by default
- Can be resource-intensive – Complex pipelines might be heavy on your database
- Harder to debug – Errors in the pipeline can be tricky to track down
Real-World Examples: Mongoose Query Syntax vs Aggregation Pipeline
Let’s look at some common scenarios and how each approach handles them:
Example 1: E-commerce Order Summary
Imagine you need to calculate total sales for each product.
Using regular queries:
// First, get all orders
const orders = await Order.find({ status: 'completed' });
// Then process them in your application
const productSales = {};
orders.forEach(order => {
const productId = order.productId.toString();
if (!productSales[productId]) {
productSales[productId] = 0;
}
productSales[productId] += order.amount;
});
// Convert to array for sorting
const salesArray = Object.entries(productSales).map(([id, amount]) => ({
productId: id,
totalSales: amount
}));
// Sort by total sales
salesArray.sort((a, b) => b.totalSales - a.totalSales);
Using aggregation:
const salesArray = await Order.aggregate([
{ $match: { status: 'completed' } },
{ $group: {
_id: '$productId',
totalSales: { $sum: '$amount' }
}},
{ $sort: { totalSales: -1 } }
]);
The aggregation version is not only shorter but also likely faster since all the processing happens in the database.
Example 2: Blog Posts with Authors and Comment Counts
Let’s say you need a list of blog posts with author details and comment counts.
Using regular queries:
// Get posts with authors
const posts = await Post.find()
.populate('author')
.lean();
// For each post, fetch the comment count
for (const post of posts) {
post.commentCount = await Comment.countDocuments({ postId: post._id });
}
Using aggregation:
const posts = await Post.aggregate([
{ $lookup: {
from: 'users',
localField: 'author',
foreignField: '_id',
as: 'authorDetails'
}},
{ $unwind: '$authorDetails' },
{ $lookup: {
from: 'comments',
localField: '_id',
foreignField: 'postId',
as: 'comments'
}},
{ $project: {
title: 1,
content: 1,
createdAt: 1,
author: '$authorDetails',
commentCount: { $size: '$comments' }
}}
]);
The regular query approach requires multiple database calls (one for posts, then one per post for comments). The aggregation pipeline gets everything in a single query.
Performance: Speed and Efficiency
When it comes to performance, things get interesting:
- For simple queries, regular
find()
operations are often faster than aggregation, especially when working with indexes. - For complex operations, aggregation usually wins because it reduces the data being transferred and processed by your application.
Here’s the general rule: the more processing you need to do after getting your data, the more likely it is that aggregation will be faster.
Key Performance Tips
- Use indexes efficiently with both approaches
- For aggregation, put your
$match
stages early in the pipeline - Use
.lean()
with regular queries when you don’t need Mongoose models - Test both approaches with your actual data if performance is critical
Readability and Maintainability: Which Is Easier to Work With?
Code that’s easy to understand is code that’s easy to maintain. Here’s how the two approaches compare:
Regular queries are generally more readable for simple operations:
User.find({ active: true })
.sort('-lastLogin')
.limit(10)
This reads almost like English: “Find active users, sort by last login date (newest first), and limit to 10 results.”
Aggregation pipelines can get lengthy, but each stage is clear in its purpose:
User.aggregate([
{ $match: { active: true } },
{ $sort: { lastLogin: -1 } },
{ $limit: 10 }
])
For complex operations, aggregation can actually be more readable because all the logic is in one place, rather than spread across your application code.
Which Approach Should You Choose?
After exploring both options, here are my recommendations:
Use regular queries when:
- Your needs are simple (basic CRUD operations)
- You’re working with a single collection
- You want to work with Mongoose models
- Your team is more familiar with this syntax
Use aggregation when:
- You need to transform data within the database
- You’re working with multiple collections at once
- You want to calculate values (sums, averages, etc.)
- You’re building reports or analytics
- You need to reduce the amount of data transferred to your app
Best Practices for Both Approaches
No matter which method you choose, keep these tips in mind:
- Start simple – Don’t use aggregation if a regular query will do
- Use proper indexes – They’re crucial for performance with both approaches
- Keep your code clean – Break complex pipelines into variables for readability
- Document your code – Especially for complex aggregation pipelines
- Test performance – If speed matters, benchmark both approaches with your data
Conclusion
Both Mongoose’s regular query syntax and the aggregation pipeline have their place in your MongoDB toolkit. Regular queries are perfect for simple operations, while aggregation shines for complex data transformations.
The good news is that you don’t have to choose just one! You can use regular queries for simple operations and switch to aggregation when you need more power. This balanced approach gives you the best of both worlds: simplicity when possible, power when necessary.
By understanding the strengths and weaknesses of each approach, you can make better decisions about how to interact with your data, leading to faster, more efficient applications.
Remember, the right tool depends on the job at hand. Sometimes the simple approach is best, and sometimes you need the heavy machinery of aggregation. Now you know when to use each one!